Laravel with React.js and Redis as a Cache
September 24, 2023
Laravel ,Technology ,web
Admin
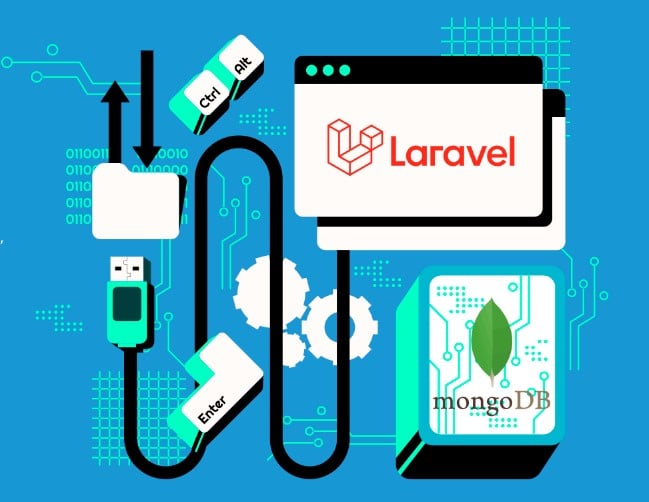
Introduction
In the ever-evolving landscape of web development, harnessing the synergy of powerful technologies is essential for building high-performance, scalable, and user-friendly applications. Laravel, a robust PHP framework, and React.js, a popular JavaScript library for building user interfaces, are two such technologies that complement each other seamlessly. In this article, we’ll explore how to use Laravel with React.js and leverage Redis as a cache to create a dynamic and efficient web application. We’ll delve into the integration process, and the benefits of this combination, and provide practical examples to guide you through the implementation.
Setting the Stage: An Overview of Laravel with React.js, and Redis
Before diving into the integration process, let’s briefly introduce the key players in this powerful combination:
Laravel: Laravel is a PHP web application framework known for its elegant syntax and developer-friendly features. It simplifies common tasks like routing, authentication, and database interactions.
React.js: React.js, developed by Facebook, is a JavaScript library for building user interfaces. It provides a component-based architecture, making it easy to create reusable UI elements.
Redis: Redis is an open-source, in-memory data store that can serve as a cache, message broker, and more. It’s exceptionally fast and suitable for handling real-time applications.
In this article, we’ll explore how to harness the strengths of Laravel and React.js while using Redis as a cache to optimize the performance of your web application. By the end, you’ll have a comprehensive understanding of how these technologies can work harmoniously to create modern and efficient web applications.
Why Combine Laravel with React.js?
The combination of Laravel and React.js offers a powerful development stack that’s becoming increasingly popular for building modern web applications. Here’s why:
Separation of Concerns: Laravel excels in handling backend logic, providing a structured and organized environment for server-side tasks. React.js, on the other hand, is a front-end powerhouse for crafting interactive user interfaces. This clear separation of concerns allows developers to focus on their respective domains, making code maintenance and collaboration more manageable.
API-Driven Development: Laravel makes it seamless to build RESTful APIs, which serve as the backbone of your application. React.js, with its component-based architecture, seamlessly consumes these APIs, creating a dynamic and responsive user experience. This approach promotes flexibility, scalability, and a smooth development workflow.
Real-Time Updates: React.js’s virtual DOM and Redis’s caching capabilities can be combined to achieve real-time updates in your application. Whether it’s chat functionality, live notifications, or dynamic data visualization, this combination enables a responsive and engaging user experience.
Performance Optimization: Redis, as an in-memory cache, significantly boosts application performance by reducing database load. This is especially beneficial for read-heavy applications or scenarios where data changes infrequently.
Community Support: Both Laravel and React.js have vibrant and active communities. This means access to extensive documentation, libraries, and a wealth of tutorials to aid in your development journey.
By bringing together the strengths of Laravel and React.js, you can build feature-rich, high-performance web applications that cater to the demands of modern users.
Installing Laravel with React.js
Setting up your development environment with Laravel with React.js is the first step toward harnessing their combined potential. Here’s how to get started:
Prerequisites:
- PHP: Ensure you have PHP installed on your machine. Laravel requires PHP 7.x or higher.
- Composer: Composer is a dependency management tool for PHP. Install it by following the instructions on the Composer website.
- Node.js and npm: To work with React.js, you’ll need Node.js and npm (Node Package Manager) installed.
Setting Up Laravel:
-
- Install Laravel: Use Composer to create a new Laravel project:
composer create-project --prefer-dist laravel/laravel project-name
-
- Serve Your Application: Navigate to your project directory and start the development server:
php artisan serve
- Access Your App: Open a web browser and go to http://localhost:8000. You should see the Laravel welcome page.
Setting Up React.js:
-
- Create a React App: Use Create React App, a popular tool for setting up React projects:
npx create-react-app react-app
-
- Start the React Development Server: Navigate to your React app directory and run:
npm start
- Access Your React App: Open a web browser and go to http://localhost:3000. You should see your React app’s homepage.
With both Laravel and React.js set up, you’re ready to dive into building the backend API and the frontend components of your application.
Building a RESTful API with Laravel
Laravel excels at creating RESTful APIs, which will serve as the bridge between the frontend React.js application and the backend data. Here’s how to build a simple RESTful API with Laravel:
-
- Creating Routes:
Define routes in Laravel’s routes/api.php file. For example:
Route::get('/items', 'ItemController@index');
Route::post('/items', 'ItemController@store');
-
- Creating Controllers:
Generate a controller using Artisan command-line tool:
php artisan make:controller ItemController
Populate the controller with methods like index() to fetch data, store() to create new records, and so on.
-
- Defining Models:
Create models using Artisan:
php artisan make:model Item
Define the model’s attributes and relationships.
-
- Database Migrations:
Create database migrations to define the database schema:
php artisan make:migration create_items_table
Modify the migration file to define table structure and relationships.
-
- Database Seeding:
Seed the database with sample data for testing and development:
php artisan make:seeder ItemSeeder
Modify the seeder file to populate the database.
-
- Using Eloquent ORM:
Leverage Laravel’s Eloquent ORM to interact with the database. For example:
// Fetch all items
$items = Item::all();
// Create a new item
$item = Item::create([‘name’ => ‘Sample Item’]);
-
- Response Formatting:
Format API responses as JSON using Laravel’s response() method.
By following these steps, you can create a robust RESTful API that React.js will interact with to retrieve and manipulate data in your application.
Creating a React.js Frontend
With your Laravel API in place, it’s time to craft a dynamic and user-friendly frontend using React.js. Here’s how to get started:
Component-Based Structure:
React.js is built around the concept of components. Design your application by breaking it down into reusable components, such as navigation bars, forms, and list views.
State Management:
Utilize React’s state management to handle dynamic data. You can use state to store and update information that changes over time, such as user input or fetched data from the API.
Component Lifecycle:
Understand the component lifecycle to control when components render and update. Lifecycle methods like componentDidMount() are essential for fetching data from your Laravel API.
Routing:
Implement client-side routing using libraries like react-router-dom. Define routes for different views and components within your application.
Handling Forms:
Create forms for user input and handle form submissions. Use React’s controlled components to manage form state.
UI Libraries and Styling:
Choose a UI library (e.g., Material-UI, Ant Design) to enhance the look and feel of your application. You can also style components using CSS, SCSS, or styled-components.
Interacting with the API:
Use JavaScript’s fetch() or libraries like Axios to make API requests to your Laravel backend. Ensure that your API calls adhere to RESTful principles.
Component Reusability:
Strive for component reusability by creating generic components that can be used in various parts of your application.
With these steps, you’ll create a well-structured React.js frontend that communicates seamlessly with your Laravel backend through the RESTful API.
Integrating Laravel and React.js
To connect the frontend React.js application with the backend Laravel API, you need to establish a smooth communication flow. Here’s how to integrate these two components:
CORS Configuration:
Cross-Origin Resource Sharing (CORS) can be a hurdle when connecting frontend and backend applications hosted on different domains. Laravel provides middleware for configuring CORS settings. You can use packages like fruitcake/larvavel-cors to simplify this process.
API Requests:
In your React components, use JavaScript’s fetch() or Axios to make HTTP requests to your Laravel API. Ensure that you provide the correct API endpoint URLs for various CRUD operations.
Authentication and Authorization:
Implement authentication and authorization mechanisms to secure your API endpoints. Laravel’s built-in Passport package can help you create OAuth2-based authentication.
Handling Responses:
Parse and handle API responses in your React components. You can use async/await or promises to manage asynchronous API calls.
Error Handling:
Implement robust error-handling mechanisms to gracefully handle errors returned by the API. Consider using global error handlers and displaying user-friendly error messages.
Testing API Calls:
Test API calls within your React application using libraries like Jest and React Testing Library. These tests ensure that API integrations work as expected.
State Management:
Update React component state with data fetched from the API. Use React’s lifecycle methods (e.g., componentDidMount()) to trigger API calls when components mount.
Real-Time Updates:
If your application requires real-time updates, consider integrating WebSocket libraries like Socket.io to enable bidirectional communication between the front end and backend.
By following these integration steps, you can create a seamless connection between your Laravel with React.js frontend, allowing for efficient data transfer and interaction.
Leveraging Redis as a Cache Laravel with React.Js:
Redis, an in-memory data store, serves as an excellent cache for improving the performance and responsiveness of your Laravel and React.js applications. Here’s why Redis is a valuable addition to this technology stack:
In-Memory Speed: Redis stores data in memory, which makes it incredibly fast for read operations. When frequently accessed data is cached in Redis, it reduces the need to fetch the same data from the database repeatedly.
Cache Expiration: Redis allows you to set expiration times for cached data. This ensures that cached data remains fresh and accurate, reducing the risk of serving outdated content to users.
Key-Value Store: Redis operates as a key-value store, making it versatile for caching various types of data, such as database query results, API responses, and session data.
Publish-Subscribe Mechanism: Redis supports a publish-subscribe mechanism, enabling real-time communication between different parts of your application.
Scalability: Redis can be configured as a distributed cache, providing scalability and redundancy for applications that need to handle high traffic loads.
In the context of a Laravel and React.js application, Redis can be used to cache API responses, database query results, and any other frequently accessed data. This significantly reduces the load on your database and speeds up data retrieval, resulting in a more responsive user experience.
In the following sections, we’ll explore how to implement Redis caching in your Laravel application and leverage it to optimize data retrieval for your React.js frontend.
Implementing Redis Cache in Laravel with React Js
Integrating Redis as a cache in your Laravel application is straightforward. Here’s how to set it up:
Install Redis:
Ensure that you have Redis installed on your server. You can download and install Redis from the official website or use package managers like apt (for Linux) or Homebrew (for macOS).
Laravel Redis Package:
Laravel provides a Redis driver out of the box. You can configure it in your .env file by specifying the Redis server’s host and port:
REDIS_HOST=127.0.0.1
REDIS_PORT=6379
Using Redis Cache:
Laravel makes it easy to cache data using Redis. For example, you can cache the result of a database query like this:
$data = Cache::remember('key', $minutes, function () {
return DB::table('table')->get();
});
Cache Expiration:
Set the cache expiration time in minutes. Cached data will automatically expire and be refreshed from the source after this period.
Cache Tags:
Laravel supports cache tags, allowing you to group related cached items and invalidate them together when needed.
Cache Invalidation:
Use Laravel’s cache invalidation methods to remove specific cached items or tags. This ensures that stale data is cleared from the cache.
Cache Prefixing:
Customize cache keys by prefixing them with a unique identifier to avoid key collisions in shared Redis instances.
By configuring Laravel to use Redis as a cache, you can seamlessly cache data, improve response times, and optimize the performance of your application. In the next section, we’ll discuss cache invalidation and expiration strategies to ensure your application remains efficient.
Cache Invalidation and Expiration Strategies
Cache management is a crucial aspect of using Redis to enhance your Laravel and React.js application’s performance. Implementing effective cache invalidation and expiration strategies ensures that your cached data remains accurate and up-to-date:
Cache Invalidation:
- Manual Invalidation: Use Laravel’s cache removal methods to manually invalidate specific cached items or tags when data changes. For example, when a user updates their profile, you can invalidate the cached user information.
- Event-Driven Invalidation: Implement event listeners and triggers to automatically invalidate cache items when related data changes. Laravel’s event system is powerful for this purpose.
Cache Expiration:
- Time-Based Expiration: Set reasonable expiration times for cached data based on the expected update frequency. For instance, if a news feed rarely changes, you can set a longer cache duration, while user session data may have a shorter duration.
- Activity-Based Expiration: Implement cache expiration based on user activity. For example, clear the cache for a user’s shopping cart when they complete a purchase.
Cache Tags:
- Grouping Related Data: Use cache tags to group related cached items. When a tag is invalidated, all cached items associated with that tag are removed, ensuring consistency.
- Nested Tags: Laravel allows nesting tags within tags, providing flexibility in organizing cached data.
Clearing All Caches:
- Fallback Mechanism: In scenarios where the cache becomes corrupted or unpredictable, implement a fallback mechanism to fetch data from the source directly.
Testing Cache Behavior:
- Unit Testing: Write unit tests to ensure that your cache invalidation and expiration strategies work as expected. Laravel’s testing tools provide robust support for this.
By carefully planning and implementing cache management strategies, you can harness the full potential of Redis as a cache to optimize the performance and responsiveness of your Laravel and React.js application.
In the next section, we’ll delve into practical use cases to demonstrate how these technologies work together to build real-world applications.
Practical Use Cases
To illustrate the power of combining Laravel, React.js, and Redis, let’s explore practical use cases where this technology stack excels:
E-commerce Platform:
Create a responsive e-commerce website using React.js for the frontend. Implement features like product catalogs, real-time inventory updates using Redis, and seamless checkout with Laravel handling payment processing.
Social Networking App:
Build a social networking platform with React.js for dynamic user interfaces. Utilize Redis for real-time notifications, friend requests, and chat functionality. Laravel can handle user authentication and API interactions.
Content Management System (CMS):
Develop a content management system with React.js for the frontend and Laravel as the backend. Implement Redis caching for frequently accessed content, such as articles and media files, to enhance page load times.
Dashboard and Analytics Tools:
Create interactive dashboards and analytics tools using React.js for data visualization. Leverage Redis for caching and efficiently retrieve large datasets. Laravel can serve as the API backend.
Real-Time Chat Application:
Build a real-time chat application using React.js for the frontend. Redis can be used to manage online status, store chat history, and facilitate instant messaging. Laravel can handle user authentication and message routing.
These use cases demonstrate the versatility and scalability of the Laravel-React.js-Redis stack, showcasing its potential to address a wide range of web development requirements.
Testing and Debugging
Effective testing and debugging are vital to maintaining the reliability and performance of your Laravel, React.js, and Redis application. Here are some key considerations:
Unit Testing:
Write unit tests for both the Laravel backend and React.js frontend. Laravel’s built-in testing tools and libraries like Jest for React make it easier to ensure that individual components and functions work as intended.
Integration Testing:
Perform integration tests to validate the interactions between different parts of your application, such as API endpoints and React components. Tools like Laravel Dusk and testing libraries like React Testing Library can help with this.
Continuous Integration (CI):
Implement CI/CD pipelines to automate testing and deployment. Services like Travis CI and GitHub Actions can be integrated with your version control system to ensure that tests are run automatically on code changes.
Debugging Tools:
Utilize debugging tools provided by Laravel, React.js, and browser developer tools. Laravel’s logging and debugging features, React DevTools, and browser consoles are valuable resources for identifying and resolving issues.
Error Tracking:
Implement error tracking and monitoring tools like Sentry or New Relic to capture and analyze application errors in real-time. This helps in identifying and addressing issues proactively.
Load Testing:
Perform load testing to assess your application’s performance under heavy traffic. Tools like Apache JMeter and Locust can simulate concurrent users to identify potential bottlenecks.
Effective testing and debugging practices are essential for maintaining the stability and reliability of your Laravel and React.js applications, especially when Redis is used to optimize performance.
Deployment Considerations
Deploying a Laravel, React.js, and Redis application requires careful planning and consideration of various factors. Here are key deployment considerations:
Server Infrastructure:
Choose a hosting environment that suits your application’s requirements. Options include traditional web hosts, cloud providers (e.g., AWS, Azure, GCP), and serverless architectures.
Scaling Strategies:
Plan for scalability. Utilize load balancing and auto-scaling features to handle increased traffic and demand. Redis can be configured for clustering in high-traffic scenarios.
Environment Configuration:
Ensure that your production environment configuration is optimized for security, performance, and reliability. Implement environment-specific settings in Laravel.
Database Backups:
Regularly backup your database to prevent data loss. Set up automated backup schedules and store backups securely.
SSL/TLS Encryption:
Enable SSL/TLS encryption to secure data transmission between clients and your server. Obtain and configure SSL certificates for your domain.
CDN Integration:
Use Content Delivery Networks (CDNs) to distribute static assets and reduce latency. CDNs improve the delivery of React.js bundles and other frontend resources.
Containerization (Optional):
Consider containerization using Docker and orchestration tools like Kubernetes for easier deployment and management of your application.
Monitoring and Logging:
Implement monitoring and logging solutions to track the health and performance of your application in production. Tools like Prometheus, Grafana, and ELK stack are popular choices.
Error Handling and Alerts:
Configure alerts and notifications for critical errors and performance anomalies. Set up alerting systems to promptly respond to issues.
Rollback Plans:
Prepare rollback plans in case of deployment failures. Maintain previous versions of your application for easy rollback.
Version Control and CI/CD:
Use version control (e.g., Git) and CI/CD pipelines to automate deployment processes and ensure consistency.
Security Considerations:
Continuously monitor and update your application’s security measures. Regularly apply security patches and follow best practices for secure coding.
By addressing these deployment considerations, you can confidently deploy your Laravel, React.js, and Redis application to a production environment while maintaining performance, security, and reliability.
Performance Optimization
Optimizing the performance of your Laravel, React.js, and Redis application is essential for delivering a seamless user experience. Here are performance optimization strategies:
Redis Cache Tuning:
Fine-tune Redis caching settings, including memory allocation and eviction policies, to optimize data retrieval and storage efficiency.
Minification and Compression:
Minify and compress frontend assets (JavaScript, CSS) to reduce load times. Use Laravel mix or webpack for this purpose.
Lazy Loading:
Implement lazy loading for images and other non-critical assets to improve initial page load times.
Code Splitting:
Utilize code splitting techniques to split your React.js application into smaller bundles, loading only the necessary code for each page.
Server-Side Rendering (SSR):
Consider server-side rendering with technologies like Next.js for React.js applications to improve SEO and initial load times.
Optimizing Database Queries:
Profile and optimize database queries using Laravel’s built-in query builder and Eloquent ORM. Implement database indexing for frequently queried columns.
Content Delivery:
Serve static assets (images, videos) through CDNs to reduce server load and improve content delivery.
HTTP/2 and HTTP/3:
Use HTTP/2 or HTTP/3 to take advantage of multiplexing and other performance-enhancing features for web requests.
Caching Strategy:
Implement server-side caching in Laravel using tools like Redis or Memcached to cache responses from the backend API.
Content Delivery:
Optimize content delivery by compressing images, leveraging browser caching, and implementing responsive design for various devices.
Database Indexing:
Ensure that your database is properly indexed to speed up query execution for frequently accessed data.
Load Balancing:
Distribute incoming traffic across multiple servers to prevent overloading and improve application scalability.
By implementing these performance optimization strategies, you can enhance the speed and responsiveness of your Laravel, React.js, and Redis application, providing an exceptional user experience.
Security Considerations
Security is paramount in web development. When using Laravel, React.js, and Redis, it’s crucial to prioritize security measures at every level. Here are key security considerations:
Authentication and Authorization:
Implement robust authentication and authorization mechanisms to ensure that only authorized users can access sensitive data and perform specific actions.
Cross-Site Scripting (XSS) Prevention:
Sanitize user input and use React’s JSX syntax to mitigate XSS vulnerabilities. Laravel’s Blade templating engine also provides built-in protection against XSS.
Cross-Site Request Forgery (CSRF) Protection:
Enable Laravel’s CSRF protection middleware to guard against CSRF attacks. Ensure that React.js follows best practices for CSRF token handling when making requests.
SQL Injection Prevention:
Use parameterized queries and Eloquent ORM for database interactions in Laravel to prevent SQL injection attacks.
Content Security Policy (CSP):
Implement CSP headers to mitigate risks associated with malicious scripts and unauthorized data loading.
Input Validation:
Validate and sanitize user input on both the frontend and backend to prevent malicious data from being processed.
API Security:
Secure your API endpoints by validating requests, rate limiting, and implementing proper authentication mechanisms, such as OAuth2 with Laravel Passport.
Session Management:
Safeguard user sessions by securely storing session data and using Laravel’s built-in session management features.
Data Encryption:
Implement encryption for sensitive data at rest and in transit using SSL/TLS certificates.
Access Control:
Restrict access to system resources and data based on user roles and permissions. Laravel’s gate and policy systems are valuable for this.
Error Handling:
Avoid exposing sensitive information in error messages. Implement custom error handling to provide minimal information in case of errors.
Monitoring and Auditing:
Continuously monitor your application for security vulnerabilities and conduct security audits periodically.
Third-Party Libraries:
Keep third-party libraries and dependencies up to date to patch known security vulnerabilities.
Regular Backups:
Maintain regular backups of your data and application code to ensure recovery in case of data breaches or data loss.
By adhering to these security best practices, you can significantly enhance the security posture of your Laravel, React.js, and Redis application and protect it from common web vulnerabilities.
Conclusion
The combination of Laravel, React.js, and Redis represents a powerful stack for modern web development. Laravel provides a robust backend framework, React.js offers a dynamic and interactive frontend, and Redis serves as a high-performance cache and data store.
By following the steps outlined in this article, from setting up the development environment to optimizing performance and ensuring security, you can harness the full potential of these technologies. The resulting web applications are capable of delivering exceptional user experiences, real-time updates, and efficient data retrieval.
Whether you’re building e-commerce platforms, social networking apps, content management systems, or any other web-based application, the Laravel-React.js-Redis stack offers scalability, flexibility, and a wealth of possibilities for creating cutting-edge, responsive, and secure web solutions.