Developing Microservices with Laravel
July 11, 2024
Laravel ,Technology
Admin
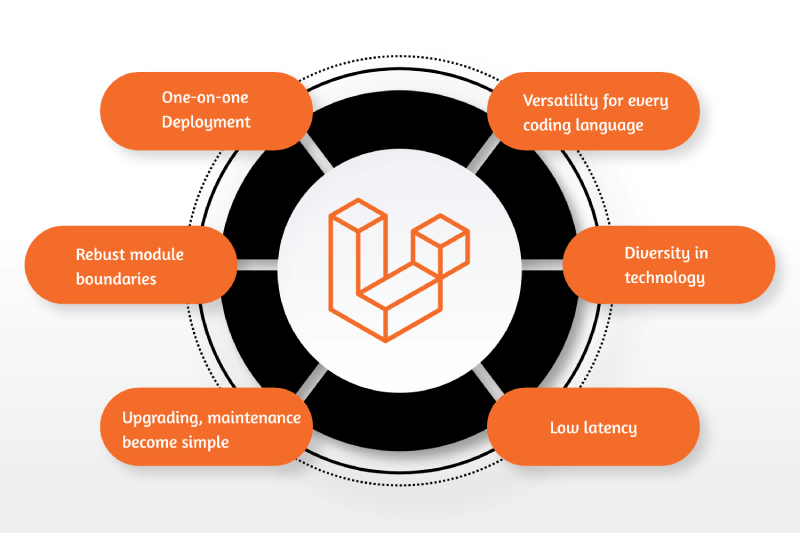
Microservices architecture has become a popular choice for building scalable and maintainable applications.
Laravel, a powerful PHP framework, offers robust features that make it an excellent choice for developing microservices. In this article, we will explore how to develop microservices with Laravel.
What are Microservices?
Microservices architecture is a design approach where a single application is composed of many loosely coupled and independently deployable services. Each service is responsible for a specific business function and communicates with other services over lightweight protocols, often HTTP or messaging queues.
Why Choose Laravel for Microservices?
- MVC Architecture: Laravel’s Model-View-Controller (MVC) architecture helps in organizing the codebase and separating business logic from presentation logic.
- Eloquent ORM: Laravel’s Eloquent ORM simplifies database interactions, making it easier to work with data.
- Artisan CLI: Laravel’s command-line interface, Artisan, provides useful commands for development and deployment tasks.
- Queue Management: Laravel offers built-in support for queues, which is essential for handling background tasks and improving application performance.
- RESTful Routing: Laravel’s routing system is designed to easily create RESTful APIs, which are essential for microservices communication.
- Middleware: Laravel’s middleware allows you to filter HTTP requests entering your application, which is crucial for security and request handling.
Setting Up Laravel for Microservices
1. Install Laravel
Start by installing Laravel using Composer:
composer create-project --prefer-dist laravel/laravel microservice-app
2. Configure Your Environment
Set up your environment by configuring the .env
file. Update the database configuration and other necessary settings.
3. Create Microservices
In a microservices architecture, each service is a separate Laravel project. For example, you might have a User Service
, Product Service
, and Order Service
.
4. Define Service Contracts
Define contracts (interfaces) for communication between services. This ensures that each service adheres to a specific contract, making integration easier.
// Contracts/UserServiceInterface.php
namespace App\Contracts;
interface UserServiceInterface {
public function getUserById($id);
public function createUser(array $data);
}
5. Implement RESTful APIs
Each microservice should expose RESTful APIs for communication. Use Laravel’s built-in routing and controller features to create APIs.
// routes/api.php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\UserController;
Route::get('/users/{id}', [UserController::class, 'show']);
Route::post('/users', [UserController::class, 'store']);
// app/Http/Controllers/UserController.php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class UserController extends Controller
{
public function show($id)
{
$user = User::find($id);
return response()->json($user);
}
public function store(Request $request)
{
$user = User::create($request->all());
return response()->json($user, 201);
}
}
6. Use Service Discovery
Service discovery is crucial for finding services in a microservices architecture. Tools like Consul or Eureka can be used for service discovery. In Laravel, you can integrate these tools using packages or custom implementations.
7. Implement API Gateway
An API Gateway acts as a reverse proxy to route requests to appropriate microservices. It also handles cross-cutting concerns like authentication, logging, and rate limiting. Tools like Kong, Zuul, or custom solutions can be used as an API Gateway.
8. Handle Inter-Service Communication
Use HTTP or messaging queues (like RabbitMQ or Kafka) for inter-service communication. Laravel’s HTTP client can be used to make HTTP requests to other services.
// app/Services/UserService.php
namespace App\Services;
use Illuminate\Support\Facades\Http;
class UserService
{
public function getUserById($id)
{
$response = Http::get('http://user-service/api/users/' . $id);
return $response->json();
}
}
9. Manage Configuration
Centralized configuration management ensures consistency across services. Tools like Spring Cloud Config or Consul can be used for this purpose.
10. Implement Logging and Monitoring
Centralized logging and monitoring are essential for managing microservices. Use tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus and Grafana for monitoring.
Example: Creating a Simple User Service
Here’s a basic example of a User Service
in Laravel:
1. Create User Model
php artisan make:model User -m
2. Update the migration file and run migrations:
// database/migrations/xxxx_xx_xx_create_users_table.php
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamps();
});
}
php artisan migrate
3. Create User Controller
php artisan make:controller UserController
4. Update the controller to handle user requests:
// app/Http/Controllers/UserController.php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class UserController extends Controller
{
public function show($id)
{
$user = User::find($id);
return response()->json($user);
}
public function store(Request $request)
{
$user = User::create($request->all());
return response()->json($user, 201);
}
}
5. Define Routes
// routes/api.php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\UserController;
Route::get('/users/{id}', [UserController::class, 'show']);
Route::post('/users', [UserController::class, 'store']);
Conclusion
Developing microservices with Laravel provides a robust and scalable solution for modern web applications. By leveraging Laravel’s powerful features and integrating with other tools and services, you can build a maintainable and efficient microservices architecture. Hire the RND Experts team to develop CRM to manage the complete sale, marketing, and production process, ensuring your microservices ecosystem is seamless and effective.